I used different fonts, some bold, some regular. The output looked really good in the iReport preview. Then I ran my application and created the PDF. All the fonts and font styles (bold, italic) were gone and had been replaced by one plain font. After googling a bit, I found the cause of the problem: the fonts I used were not embedded in the generated PDF, so they could not be shown correctly.
I will now explain how you can embed fonts in your PDF. The methods described here work on JasperReports 3.7 and greater, so make sure you have a recent version.
There are two methods to embed the fonts in your PDF. Both methods yield the same result, but one method requires the use of iReport. I will first explain the method without iReport, then the method with iReport. If you use iReport, I strongly suggest using the method with iReport, since it is much easier.
1. No iReport (the hard way)
We will use a JasperReports extension, called the simple font extension. This extension registers the necessary fonts with JasperReports so it can embed them in a PDF when needed. The first thing you need to do is to create a file called
jasperreports_extension.properties
. This file should have the following content (I broke up the lines so they fit the screen, don't do this yourself):
net.sf.jasperreports.extension.registry.factory.fonts=
net.sf.jasperreports.engine.fonts.SimpleFontExtensionsRegistryFactory
net.sf.jasperreports.extension.simple.font.families.myfamily=
fonts/myfamily.xml
Then you'll need a file called
myfamily.xml
in a fonts
folder. This is what the content should look like:<?xml version="1.0" encoding="UTF-8"?> <fontFamilies> <fontFamily name="Arial"> <normal><![CDATA[fonts/arial.ttf]]></normal> <bold><![CDATA[fonts/arialbd.ttf]]></bold> <italic><![CDATA[fonts/ariali.ttf]]></italic> <boldItalic><![CDATA[fonts/arialbi.ttf]]></boldItalic> <pdfEmbedded><![CDATA[true]]></pdfEmbedded> </fontFamily> <fontFamily name="Tahoma"> <normal><![CDATA[fonts/tahoma.ttf]]></normal> <bold><![CDATA[fonts/tahomabd.ttf]]></bold> <pdfEmbedded><![CDATA[true]]></pdfEmbedded> </fontFamily> </fontFamilies>
As you can see, I embedded two fonts here: Arial and Tahoma. Arial has a regular, bold and italic variant, while Tahoma lacks the italic variant. I set both
pdfEmbedded
to true
, to make sure they get embedded into pdfs. You will of course also have to copy the ttf files to the fonts
directory you created earlier. You will have to locate the ttf files on your harddisk. For windows, they are located in \windows\fonts
.Now all you need to do is add all the files we created and copied to the classpath of your application. To sum it up, you'll have the following files on your classpath:
jasperreports_extension.properties
: This file needs to go in the root of your classpath (so not in a package). It will be automatically picked up by JasperReports.fonts/myfamily.xml
: The xml file defining the fonts. It should be named as you declared it in the .properties file.fonts/*.ttf
: All the ttf files you reference in your xml file
2. Using iReport (the easy way)
Everything we did in the first method can be automated using iReport. The first thing you need to do is make sure the all the fonts you used in your report have been defined in iReport. You can do this by starting iReport and going to Tools -> Options -> iReport -> Fonts. You will see the following window:
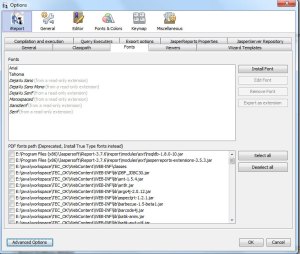
If the fonts you used are not yet installed (they probably aren't), you'll need to install them. Click the "Install Font" button. A wizard will pop up, asking you the font name and the location of the ttf files. Just direct the application to the location of the ttf files (for windows systems this is generally in the
\windows\fonts
folder). Make sure to check the "Embed this font in PDF" checkbox on the second page! All other settings can be left at their defaults. Once all the fonts are installed, you select all the fonts you used in your report (hold ctrl
to select multiple entries from the list). Then you click the "Export as extension" button. This will create a jar file. All you need to do is add the created jar file to the classpath of your application and you're done!
Good article. I think font extensions are not understood or used as well as they should be, so it's nice to see things like this.
ReplyDeleteBy the way, there is no "s" in iReport.
Thanks, that article saved my day! I was searching arround for days and did not find a suitable solution to this Problem. I could not beleive that a Report tool like Jasper cannot handle bold fonts.
ReplyDeleteHi Christoph,
ReplyDeleteAs you can see Jasper Reports can handle bold fonts, you just have to know how to configure it :-). That's the main problem with Jasper Reports: the documentation. There is the ultimate guide that's very good, but unfortunately it's not free. If you don't use Jasper Reports commercially you're on your own.
Thanks a lot. I finally made it work!
ReplyDeleteI have tried both methods, but they are only applicable if you are using Java 1.5 while I am using Java 1.4. Do we have other way to resolve this issue?
ReplyDeleteHi Andy,
ReplyDeleteFirst of all I would definitely recommend upgrading to a newer java version: 1.4 is starting to get pretty old. I suppose you don't have a choice or you would already have done so.
Can you tell me exactly which part doesn't work on java 1.4? Is it the font extension, iReport, JasperReport...? You could try mapping the fonts directly in your report xml file, as follows:
<font fontName="My Font" ispdfembedded="true"
pdffontname="path/to/myfont.ttf"/>
I haven't tried this though, so I don't know if it will work...
Hi,
ReplyDeleteI have created the fonts jar file and kept in the classpath.
After this do we need to do any changes in the jrxml file like setting "PDFembedded = true"..please clarify
Regards,
Hema
hi,
ReplyDeletemy concern is how can we make sure that the jasper report is using the fonts from fonts jar file or from windows/unix environment.
Please clarify..
Thanks in advance,
Hema.
Hi,
ReplyDeleteJasper reports will not pick up the fonts from the windows/unix environment. This is the reason you need the font extension. iReport DOES pick up the fonts from the OS, and that's the reason your report looks ok in iReport but not in JasperReport.
In the fonts.jar file is a file called jasperreports_extension.properties. Jasper reports will automatically pick up this file and read it out. It will the find your font definition file (the fontfamily.xml file or whatever you called it) and get the fonts from that file. It will all be automatic, all you need to do is add the jar file to your classpath.
Hi,
ReplyDeleteYou don't have to modify your jrxml file if you use the font extension, that was the old approach. The new approach is to use the font extension. All you have to do is make sure that the font name used in your jrxml file is the same as the one you define in the fontfamily.xml file.
Hi !! i have a question, i generate the archive.jar with fonts that i needed, y my projects i add, but i dont see this changes, and makes a error... only add .jar or i have to do something else?
ReplyDeleteHi Pollet,
ReplyDeleteWhat error are you getting? Please make sure you use a real font (Arial, Times New Roman...) and not a Java substitute font (like SansSerif)
GRAVE: java.lang.NoClassDefFoundError: org/springframework/core/io/Resource
ReplyDeleteat java.lang.Class.forName0(Native Method)
at java.lang.Class.forName(Class.java:247)
at net.sf.jasperreports.engine.util.JRClassLoader.loadClassForRealName(JRClassLoader.java:157)
at net.sf.jasperreports.engine.util.JRClassLoader.loadClassForName(JRClassLoader.java:115)
at net.sf.jasperreports.engine.util.ClassUtils.instantiateClass(ClassUtils.java:53)
at net.sf.jasperreports.extensions.DefaultExtensionsRegistry.instantiateRegistry(DefaultExtensionsRegistry.java:198)
at net.sf.jasperreports.extensions.DefaultExtensionsRegistry.loadRegistries(DefaultExtensionsRegistry.java:175)
at net.sf.jasperreports.extensions.DefaultExtensionsRegistry.loadRegistries(DefaultExtensionsRegistry.java:135)
at net.sf.jasperreports.extensions.DefaultExtensionsRegistry.getRegistries(DefaultExtensionsRegistry.java:121)
at net.sf.jasperreports.extensions.DefaultExtensionsRegistry.getExtensions(DefaultExtensionsRegistry.java:98)
at net.sf.jasperreports.engine.util.JRStyledTextParser.(JRStyledTextParser.java:76)
at net.sf.jasperreports.engine.fill.JRBaseFiller.(JRBaseFiller.java:174)
at net.sf.jasperreports.engine.fill.JRVerticalFiller.(JRVerticalFiller.java:74)
at net.sf.jasperreports.engine.fill.JRVerticalFiller.(JRVerticalFiller.java:56)
at net.sf.jasperreports.engine.fill.JRFiller.createFiller(JRFiller.java:143)
at net.sf.jasperreports.engine.fill.JRFiller.fillReport(JRFiller.java:79)
at net.sf.jasperreports.engine.JasperFillManager.fillReport(JasperFillManager.java:624)
at net.sf.jasperreports.engine.JasperFillManager.fillReport(JasperFillManager.java:540)
this is my error, so I question you, if no is necesary to do some extra thing
of course, is arial, and variations of arial
ReplyDelete(bold, italic and bold-italic)
Hi Polet,
ReplyDeleteAdd the spring-core-x.x.x.jar and spring-beans-x.x.x.jar to your application. These are needed by the font extension.
Great!!!, thank you n_n
ReplyDeletesome thing and no more, I need to see the align (left, right o justify )in the .pdf and no give it format in the ireport, i need give it format from java-jasperreport, because I receive a text in html and should respect all formats in the pdf. some idea???
ReplyDeleteHi Polet,
ReplyDeleteIf you want to pass html, just add a textfield to your report and set the "markup" property of the textfield to "html" using iReport.
Excelent!!!!
ReplyDeleteI'm using windows to compile reports than will be generated dinamicaly on linux JVM, this is better solution than install ms-core-font.
Thank you.
thxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx
ReplyDelete[net.sf.jasperreports.extensions.DefaultExtensionsRegistry] Error instantiating extensions registry for chart.theme: org.springframework.beans.factory.BeanDefinitionStoreException: Unexpected exception parsing XML document from URL [vfs:/C:/jboss-6.1.0.Final/server/default/deploy/ReqTrace.war/WEB-INF/lib/jasperreports-chart-themes-4.0.1.jar/net/sf/jasperreports/chartthemes/spring/beans/chartThemesBeans.xml]; nested exception is java.lang.IllegalArgumentException: Class [org.apache.cxf.ws.policy.spring.PolicyNamespaceHandler] does not implement the NamespaceHandler interface
ReplyDeletehey.
DeleteHow did you solve this?
Thank you
iReport 4.1.1 says error access denied when trying to install a font.
ReplyDeleteAnd manually, what should be the place to jasperreports_extension.properties? Grail app root?
If you use Windows, maybe you have Jaspersoft installed in C:/Documents and Settings/Jaspersoft/..., try reinstall the application out of the folder, like C:/Jaspersoft/... or in other path.
DeleteIt works great for me, i only added Arial Unicode MS, set PDF embedded, and now i can export in various languajes. Thank you men!
ReplyDeleteYou can try printing this for testing purposes:
Test Arabic characters - ﺀ ﻻ للها لله ﻼ ﷲ گ ﭪﭖ
Test Chinese characters - 甲 骨 文 章 草 新 隸 體 書
Test Arabic characters - ﺀ ﻻ للها لله ﻼ ﷲ گ ﭪﭖ
Test Hindi characters - अ आ इ ई उ ऊ ऋ ऌ ऍ ऎ ए ऐ
Test Russian characters - И з м е н и т ь з а д а ч и
Test Farsi characters - ماموریت نگهداری نرخ تبدیل ارز
Test Hungarian characters - Üzenet szöveg
Test Turkish characters - Açıklama
Test Spanish characters - Descripción
Test English characters - Description
Hi ,
DeleteAm trying to load a arabic text but it is loading like this "Ø§ÙØ±Ø¬Ø§Ø¡ Ø¥Ø¯Ø®Ø§Ù Ø§ÙØ¹Ø¯Ø¯ Ø§ÙØ¨Ø± Ù" any help? i have loaded the fonts and steps mentioned above.
Thanks
add the created jar file to the classpath of your application, where is classpath?
ReplyDeleteHi Cuinetes,
ReplyDeleteIt looks like you're having some problems with the classpath. I've never written a Grails applications, so I'm not sure how it works. You need to add the jar to the classpath. For web applications this is typically in the WEB-INF\lib directory. I'm not sure this is also the case for Grails application. Try locating the other .jar files in your application and place the fonts jar together with the other jar files. Then restart the server.
Hi Cuinetes,
ReplyDeletePlease follow the below link to add font extensions to Grails App
http://stackoverflow.com/questions/6711480/including-liberation-ttf-fonts-in-a-grails-war
Regards,
S.S. Khan
great article thank you,
ReplyDeleteim using jasper reports in web application pdf exporting
with above settings i wanted to embed the font
i use following font tag in jrxml file
font fontName="Arial" size="10" isItalic="false" isPdfEmbedded ="true" pdfEncoding ="Identity-H"
I am new to iReport. I ve tried to follow ur solution but faced two problems:
ReplyDelete1- When I try to intsall the font, I get a "Access deined on the font file" error , although the file is not protected and i ve even copied it to the iReports font folder.
2- The "export as extension " button in my iReport is disabled and I dont know why.
Thanks. it works.
ReplyDeleteERROR [net.sf.jasperreports.extensions.DefaultExtensionsRegistry] Error instantiating extensions registry for chart.theme from vfszip:My-App.ear/lib/jasperreports-chart-themes-4.7.0.jar/jasperreports_extension.properties
ReplyDeleteorg.springframework.beans.factory.parsing.BeanDefinitionParsingException: Configuration problem: Failed to import bean definitions from relative location [defaultChartPropertiesBean.xml]
Offending resource: URL [My-App.ear/lib/jasperreports-chart-themes-4.7.0.jar/net/sf/jasperreports/chartthemes/spring/beans/chartThemesBeans.xml]; nested exception is org.springframework.beans.factory.parsing.BeanDefinitionParsingException: Configuration problem: Failed to import bean definitions from relative location [chartConstantsBean.xml]
Offending resource: URL [My-App.ear/lib/jasperreports-chart-themes-4.7.0.jar/net/sf/jasperreports/chartthemes/spring/beans/defaultChartPropertiesBean.xml]; nested exception is org.springframework.beans.factory.BeanDefinitionStoreException: Unexpected exception parsing XML document from URL [My-App.ear/lib/jasperreports-chart-themes-4.7.0.jar/net/sf/jasperreports/chartthemes/spring/beans/chartConstantsBean.xml]; nested exception is org.springframework.beans.FatalBeanException: Class [org.springframework.beans.factory.xml.UtilNamespaceHandler] for namespace [http://www.springframework.org/schema/util] does not implement the [org.springframework.beans.factory.xml.NamespaceHandler] interface
at org.springframework.beans.factory.parsing.FailFastProblemReporter.error(FailFastProblemReporter.java:68)
Anyone can help me please?
Thanks
OK Problem Solved.
DeleteI included jasperreports-chart-themes-4.7.0.jar in my classpath. However, this jar wasn't used anymore. I only used the default themes from jasper.
Deleting this jar from .ear and everything works fine.
Thanks anyway
net.sf.jasperreports.engine.util.JRFontNotFoundException: Font '’Arial’' is not available to the JVM. See the Javadoc for more details.
ReplyDeleteat net.sf.jasperreports.engine.util.JRFontUtil.checkAwtFont(JRFontUtil.java:358)
at net.sf.jasperreports.engine.util.JRStyledText.getAwtAttributedString(JRStyledText.java:226)
at net.sf.jasperreports.engine.fill.TextMeasurer.measure(TextMeasurer.java:326)
at net.sf.jasperreports.engine.fill.JRFillTextElement.chopTextElement(JRFillTextElement.java:511)
at net.sf.jasperreports.engine.fill.JRFillTextField.prepare(JRFillTextField.java:593)
at net.sf.jasperreports.engine.fill.JRFillElementContainer.prepareElements(JRFillElementContainer.java:328)
at net.sf.jasperreports.engine.fill.JRFillBand.fill(JRFillBand.java:388)
at net.sf.jasperreports.engine.fill.JRFillBand.fill(JRFillBand.java:347)
at net.sf.jasperreports.engine.fill.JRVerticalFiller.fillTitle(JRVerticalFiller.java:329)
at net.sf.jasperreports.engine.fill.JRVerticalFiller.fillReportStart(JRVerticalFiller.java:263)
at net.sf.jasperreports.engine.fill.JRVerticalFiller.fillReport(JRVerticalFiller.java:129)
at net.sf.jasperreports.engine.fill.JRBaseFiller.fill(JRBaseFiller.java:903)
at net.sf.jasperreports.engine.fill.JRBaseFiller.fill(JRBaseFiller.java:832)
at net.sf.jasperreports.engine.fill.JRFiller.fillReport(JRFiller.java:84)
at net.sf.jasperreports.engine.JasperFillManager.fillReport(JasperFillManager.java:624)
at net.sf.jasperreports.engine.JasperRunManager.runReportToPdf(JasperRunManager.java:421)
at org.apache.jsp.jsp.print_005fexecsummary_005fjasper_jsp._jspService(print_005fexecsummary_005fjasper_jsp.java:388)
at org.apache.jasper.runtime.HttpJspBase.service(HttpJspBase.java:70)
at javax.servlet.http.HttpServlet.service(HttpServlet.java:722)
at org.apache.jasper.servlet.JspServletWrapper.service(JspServletWrapper.java:432)
at org.apache.jasper.servlet.JspServlet.serviceJspFile(JspServlet.java:390)
at org.apache.jasper.servlet.JspServlet.service(JspServlet.java:334)
at javax.servlet.http.HttpServlet.service(HttpServlet.java:722)
at org.apache.catalina.core.ApplicationFilterChain.internalDoFilter(ApplicationFilterChain.java:305)
at org.apache.catalina.core.ApplicationFilterChain.doFilter(ApplicationFilterChain.java:210)
at org.apache.catalina.core.StandardWrapperValve.invoke(StandardWrapperValve.java:222)
at org.apache.catalina.core.StandardContextValve.invoke(StandardContextValve.java:123)
at org.apache.catalina.authenticator.AuthenticatorBase.invoke(AuthenticatorBase.java:472)
at org.apache.catalina.core.StandardHostValve.invoke(StandardHostValve.java:168)
at org.apache.catalina.valves.ErrorReportValve.invoke(ErrorReportValve.java:99)
at org.apache.catalina.valves.AccessLogValve.invoke(AccessLogValve.java:929)
at org.apache.catalina.core.StandardEngineValve.invoke(StandardEngineValve.java:118)
at org.apache.catalina.connector.CoyoteAdapter.service(CoyoteAdapter.java:407)
at org.apache.coyote.http11.AbstractHttp11Processor.process(AbstractHttp11Processor.java:1002)
at org.apache.coyote.AbstractProtocol$AbstractConnectionHandler.process(AbstractProtocol.java:585)
at org.apache.tomcat.util.net.JIoEndpoint$SocketProcessor.run(JIoEndpoint.java:312)
at java.util.concurrent.ThreadPoolExecutor$Worker.runTask(Unknown Source)
at java.util.concurrent.ThreadPoolExecutor$Worker.run(Unknown Source)
at java.lang.Thread.run(Unknown Source)
please help.. i followed your steps.. its still not working
Hi Kiran,
ReplyDeleteAre you sure you added all the files to the classpath? How are you testing your application?
This comment has been removed by the author.
ReplyDeletePermiss denied when install the font... any ideas?
ReplyDeleteSolved: sudo chmod 777 to /usr/lib/iReport.4..1/ireport/fonts and restart the server.
ReplyDeleteMerci beaucoup.
ReplyDeleteHi ,
ReplyDeleteAm trying to load a arabic text but it is loading like this "Ø§ÙØ±Ø¬Ø§Ø¡ Ø¥Ø¯Ø®Ø§Ù Ø§ÙØ¹Ø¯Ø¯ Ø§ÙØ¨Ø± Ù" any help?
Hi sri,
ReplyDeleteMake sure you are using a unicode font and that it is embedded in the pdf
This comment has been removed by the author.
DeleteHi Mathias,
DeleteCan u explain about how to do it i have done this earlier but still it is not working. if possible a step by step explanation would be very helpful.
Thanks In Advance
This comment has been removed by the author.
DeleteHi Mathias,
DeleteResourceBundle bundle = ResourceBundle.getBundle("Arabic-Report", Locale.getDefault(), "Path of that Arabic-Report file");
In that bundle variable arabic text are read like this "Ø¥Ø¯Ø®Ø§Ù Ø§ÙØ¹Ø¯Ø¯ Ø§ÙØ¨Ø± Ù".
Arabic-Report is a .property(saved as UTF-8 format) file which contains key and its corresponding arabic value.
Any suggestion ?
Thanks
Hi,
ReplyDeleteI got the following error when Export as extension button is click.
"duplicate entry: fonts/Zawgyi-One-20051130.ttf"
Any idea?
Thank
Sorry, I know why. It's because I also choose that font for bold and italic.
Deletevery very helpful article ....
ReplyDeletepdf is generating in hindi in ireport tool bt when i use java code to generate pdf by passing the same jrxml,it is nt working??? :(
ReplyDeleteVery helpful. Thank you
ReplyDeletetrying to add a √ (check mark) in ireport. It displays in the ireport when run but comes blank when run from application pdf format. So which font to install for this.
ReplyDeleteThanks
It already works fine in the PDF output of the iReport, but when I used it in my Java application, the texts are not in BOLD. I already successfully made a JAR file and added it to my classpath, but still the texts are not in BOLD. I am using Arial font and would like to see some texts in bold. I am just running a local Java Application in a runnable JAR. I added the JAR via Build Path> Configure Build Path> Add JAR in Libraries Tab. Any input would help. Thanks!
ReplyDeleterap beats for saleI conclude I have selected the smart and inconceivable website along with interesting stuff.
ReplyDeletehey Mathias,
ReplyDeleteyou made my day today, every time i was suffering from server to server deployment and the font and style got changed to its default font configuration.
this is gr8 help to me.
10x !!!!
hi, this was working fine, but since a pair of days i have troubles, i get an error with the fonts in the xml file . Is there another method for get the fonts from the xml file?
ReplyDeletenet.sf.jasperreports.engine.util.JRFontNotFoundException: Font 'DejaVu Sans' is not available to the JVM. See the Javadoc for more details.
Rubén, this probably means that the DejaVu Sans font is not installed on the machine on which the report is being generated. Try installing the font on that machine.
Deletesolved. I was ussing the font extensions jar provided by jasper, i change it by another jar generated since i report and with the spring.jar fle works
ReplyDeletei dont know if you could help me plz, i have this error message in glassfish when i try to print a ireport in a web page (in pdf format):
ReplyDeletenet.sf.jasperreports.engine.util.JRFontNotFoundException: Font '
net/sf/jasperreports/fonts/pictonic/pictonic.ttf
net/sf/jasperreports/fonts/pictonic/pictonic.svg
net/sf/jasperreports/fonts/pictonic/pictonic.eot
net/sf/jasperreports/fonts/pictonic/pictonic
the thing is that pictonic isnt one of the .ttf in Windows/fonts, it is just a Font in ireport and im not even using in in my reports... i havent changed fonts, everything is in SansSerif, im using ireport 5.6 and netbeans 8.0 in Windows 7, ill thank any help.
P.S: sorry for my english :)
if anyone get the same error i`ve had, i`ve solved it by changing from iReport 5.6 to iReport 5.2
ReplyDeleteHi everybody.
ReplyDeleteI'm using Dynamicreports. I guess configuring fonts in dynamicreports and jasperreports are the same :)
1)I create a maven java application project (drFonts-project) using (dynamicreports-core 4.0.0) as dependency.
In this project, i create a myfonts.xm, a jasperreports_extension.properties and a dynamicreports-defaults.xml files in the
resources folder. I then copy all the .ttf files in a package and configure all.
2) I create other maven application (dr-test-project) using the above (drFonts-project) as dependency and create a test class in other to test report using dynamicreports.
When running the test class in Netbeans, everything seems ok (the report launch well), the default font and size configured in the dynamicreports-defaults.xml file work correctely.
But when packaging the dr-test-project in a .JAR file and try to run the dr-test-project.JAR, this error occured :
net.sf.jasperreports.engine.JRException: Input stream not found at fonts.xml.
What can I do? It seem that another file named fonts.xml is somewhere but my fonts files is myfonts.xml.
ok
DeleteI have same problem with PHP language, When I export chinese content to PDF error font. I am using libaray mPDF
ReplyDeleteThank you so much for providing step by step approach to solve this issue.
ReplyDeleteHi, I've followed step by step and I am still getting JRRuntime exception could not load font. I have the font installed already. Plse help
ReplyDeleteThis comment has been removed by the author.
ReplyDeleteThat's probably the most important step. I don't know your application nor your build process, but the files need to end up in the classpath. For a web application that's in the WEB-INF/lib, for a desktop application you will need to add them to the MANIFEST
DeleteThis comment has been removed by the author.
DeleteHey Mathias, you are correct. It works fine but if I try to use the font extension to specify a default that is used for all jasper reports, it does not work.it can't find it.
ReplyDeleteIf I use any of the fonts that appear under the top section of the iReport screen shot shown in this thread (Arial, Tahoma, ...., Serif, SanSerif), do these work by default with jasper reports? or do I still have to build an extension and add it to the class path?
ReplyDeleteYou will probably still need the extension
DeleteHey ,
ReplyDeletePlease help me with this i did this second method but after clicking on export to extension button a window opens to add jars file path but i don't know which jar files to add and how to identify them?
When you click "export to extension", the application will create a jar file. You can give it any name and location you want. Then you will need to add the generated jar file to the classpath of your application (how to do this depends on the type of application and your build system).
DeleteI did the same thing but still pdf is not generating in hindi font in jasper iReport tool.Please help for the next.
DeleteI did the same thing but still pdf is not generating in hindi font in jasper iReport tool.Please help for the next.
ReplyDeleteIf it doesn't work in iReport then you have a different problem than the one in this article. What data source are you using to test your report? Make sure the data source is UTF-8 and has correct Hindi data in it.
DeleteThis comment has been removed by the author.
ReplyDeleteHello Sir Mathias,
ReplyDeleteI'm currently working with iReport 4.6.O.
Your tutorial looks very helpful.
I could get the required jar for "Berlin Sans FB" font and I putted it in the classpath of my project.
But it can't work for me.
Could you please tell me what's can be missed ?.
Thanks for advance.
This comment has been removed by the author.
ReplyDeleteHi Sir Mathias,
ReplyDeleteIt was solved for me !.
The jar should be putten on the different classpaths on the project not only under the lib folder.
Thanks for your help.
Glad you got it to work!
DeleteHi
ReplyDeleteI am using jasperreport in the spring MVC. when I create the jasperreports_extension.properties already I don't know where to create it in my project, and with the font too. because it is not working when I put it in the /webapp. Could you help me where to put it in my project.
there is a message when i trying to install font: access denied.
ReplyDeletedavidprasetyo.com
helo
ReplyDeletei have problem with embedded font, when i run ireport and export to pdf in pdf properyty i have embedded fond but when i run in java program i have other font 'Helvetica' not embedded why.
i add .jar with instal fonts in ireport
thanks a lot for your share..
ReplyDeletethanks a lot for your share..
ReplyDeleteHello, How can I display the Japanese, Chinese, Korean, Russian languages in a pdf report for jasper reports using "Tahoma" font?
ReplyDeleteThis comment has been removed by the author.
ReplyDeleteHi Mathias,
ReplyDeleteI embedded fonts into jasperreports-fonts-4.5.0.jar and placed this under lib folder. however when i try to print my pdf, its empty.
1> Is it problem with jar misplaced?
2> whether the font is not installed in machine?
Hi rams,
DeleteI don't think this is a font issue. If the font is missing, jasper reports uses a default font. If the report is empty, there is probably an error somewhere. Start by looking in your logs and/or traces.
Hi,
ReplyDeleteWith the font family Arial Unicode MS it is working, when i changed the font family to DejaVu Sans and i am unable to get the Chinese characters.
I want to print the report in PDF for font family as DejaVu Sans.
Please help me out.
hello!
ReplyDeleteis there a sample project to see?
ty!
ReplyDeleteدلار
Please Click Above Link here & Get information aboutدلار
.
This comment has been removed by the author.
ReplyDeleteIs this works on iReport 5.6.0 too? I tried but not succeeded.
ReplyDeleteI'm using table modal data source and trying to show Sinhala. Working on swing application.
Report works as expected in jasper Viewer. But when I export, It's not.
This is how I export a pdf.
JasperExportManager.exportReportToPdfFile(jasperPrint, path);
Any idea what am I missing?
Found something myself,
DeleteI added some jar files, as noticed in the box in bottom of (Tools->Options-> Font) window.
1.hsqldb-1.8.0-10.jar
2.jasperreports-core-renderer.jar
3.jasperreports-extensions-3.5.3.jar
To class path and, Set the PDF encoding to : Identity-H (Unicode with horizontal writing).
Hope this will save someones job like mine :D
Tanks.
How to use same of "sans-serif" font family.if i'm using then getting Exception.please give me some solution.
ReplyDeleteThank you so much for this article, recommended by
ReplyDelete"David Rekowski's random stuff", helped me a lot!!
Thanks alot.. I appreciate..
ReplyDeletenice sharing
ReplyDeleteDafont.com is a site where you can download a ton of free fonts. You can search for a specific typeface, or search by the type of lettering you want, whether it’s serif or sans serif, hand lettered or grunge style. You can also put in your own phrase to see how it looks in a particular font. A lot of these fonts are very decorative and many are handdrawn, so it’s not always the best place to search for body text fonts. Each selection also tells you whether your download is free for personal or commercial use. The download is easy – you get a zip file with the font file inside. Unzip, install, and you’re ready to go.
ReplyDelete